Automating my standing desk to make me stand more
Using a Raspberry Pi to randomly pick times during my work day to change my desk to standing mode
Just like workout equipment, we all start with good intentions when getting a standing desk. As many other people did during the pandemic, I got myself a standing desk after making the switch to working from home.
Sadly, even with a brand new standing desk, I still found myself sitting for long periods. It’s not that I didn’t want to stand. Often, I would tell myself I would stand later, or just completely forget that I had a standing desk when focused in on a project or task.
Finding a solution
Once I accepted that I wasn’t standing as much as I should be, even with a new standing desk. I went on the search to see if it was possible to automate it. My goal was to remove as much friction as possible. Unfortunately, the standing desk I had didn’t have any options to achieve that.
A handful of Google search’s later. I found an example of someone tackling automating their standing desk[1]. This was perfect because I already had most of the parts I needed!
Parts used
-
Electric Standing Desk
- Raspberry Pi (I used a Model B that was collecting dust)
- Relay
How it works
For my standing desk, the easiest way I found to get what I wanted was to wire in the relay and Raspberry Pi to the pre-set height button. This way, it was simple enough to trigger the relay to “press” my standing height button.
Here is some test Python code that turns on an LED with the Raspberry Pi by powering the pin for the relay.
# test.py
import time
from gpiozero import LED
# GPIO pin 17
led = LED(17)
# Should turn relay on for 1s
led.on()
time.sleep(1)
led.off()
time.sleep(1)
Once I had the relay working with some test code I could move forward with soldering it directly into my standing desk control board. That way I could press the button via the Raspberry Pi with a Python script.
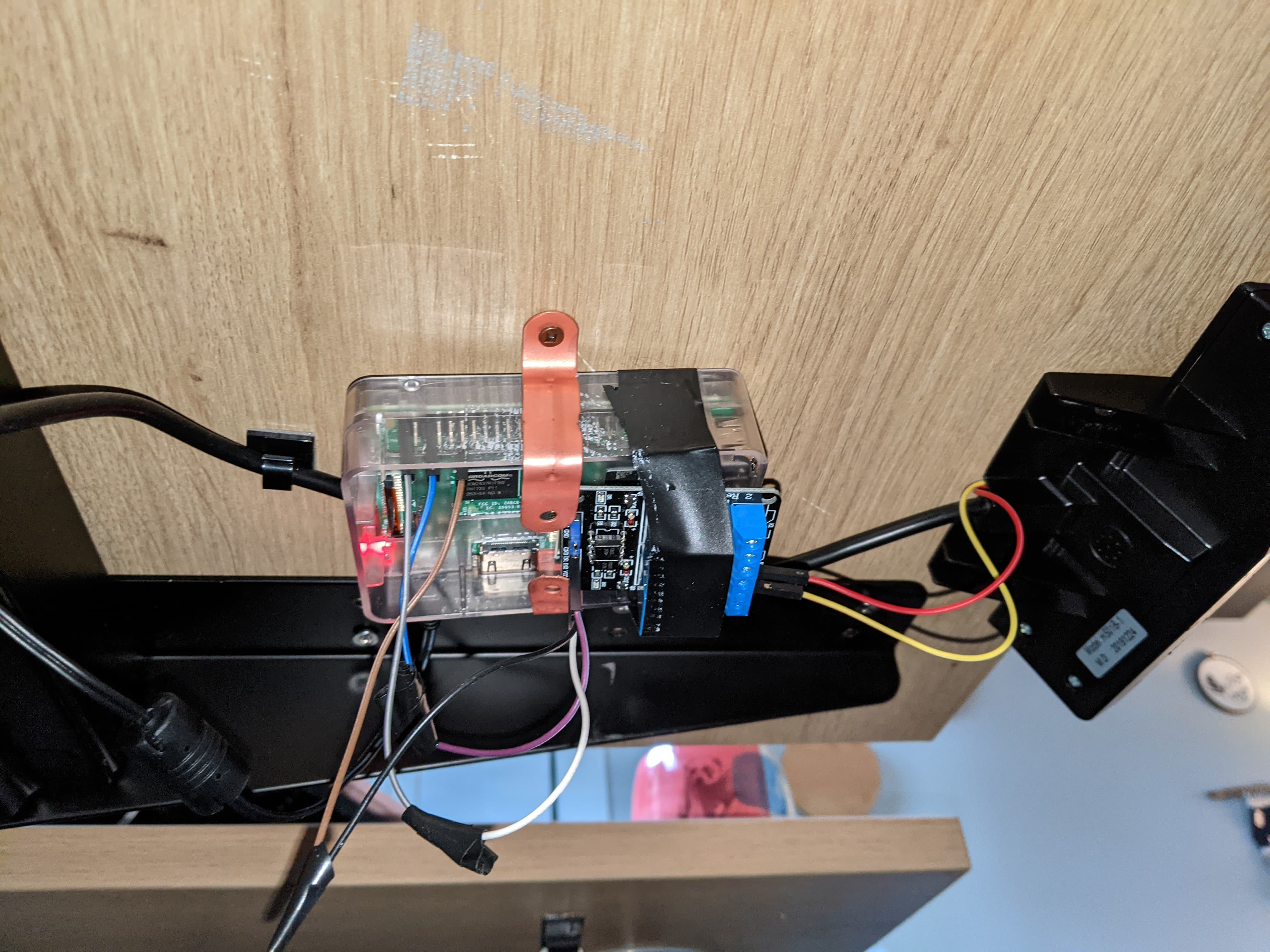
Not the most aesthetically pleasing setup but at least its hidden under a desk.
Now for the Python script that sets up random times each day and clears themselves from the schedule once ran.
# auto-standing-desk.py
from gpiozero import LED
from random import randint, choice
from time import sleep
import schedule
led = LED(17)
led.on() # on == off
# Switches pin off for 1s
def switchOn():
led.off() # off == on
sleep(1)
led.on() # on == off
# Makes desk stand up and then cancels the job
def standUp():
print("Time to stand up!")
switchOn()
return schedule.CancelJob
# Generates 2 random hour:minutes and schedules the jobs
def setupStandTimes():
randomHourOne = randint(10,11)
randomMinOne = randint(1,59)
randomHourTwo = randint(13,16)
randomMinTwo = randint(1,59)
randomTimeOne = f"{str(randomHourOne).zfill(2)}:{str(randomMinOne).zfill(2)}"
randomTimeTwo = f"{str(randomHourTwo).zfill(2)}:{str(randomMinTwo).zfill(2)}"
jobOne = schedule.every().day.at(randomTimeOne).do(standUp)
jobTwo = schedule.every().day.at(randomTimeTwo).do(standUp)
print(f"Will stand desk up at {randomTimeOne} and {randomTimeTwo} today")
return jobOne, jobTwo
# Generate new times at 8am on M,T,W,T,F
schedule.every().monday.at("08:00").do(setupStandTimes)
schedule.every().tuesday.at("08:00").do(setupStandTimes)
schedule.every().wednesday.at("08:00").do(setupStandTimes)
schedule.every().thursday.at("08:00").do(setupStandTimes)
schedule.every().friday.at("08:00").do(setupStandTimes)
while True:
schedule.run_pending()
sleep(1)
Now you can run the Python script on your Raspberry Pi and it will randomly pick times during the day to stand up.
Github Repo
Update: I’ve been using this automated standing desk setup for 2 years and it works great! Not going to lie though… I often stop it from going to standing mode by manually pressing the lower preset button. I may need to figure out how to disable all the other buttons for a certain amount of time so I have no choice but to stand. That’s a project for another day though 🤷